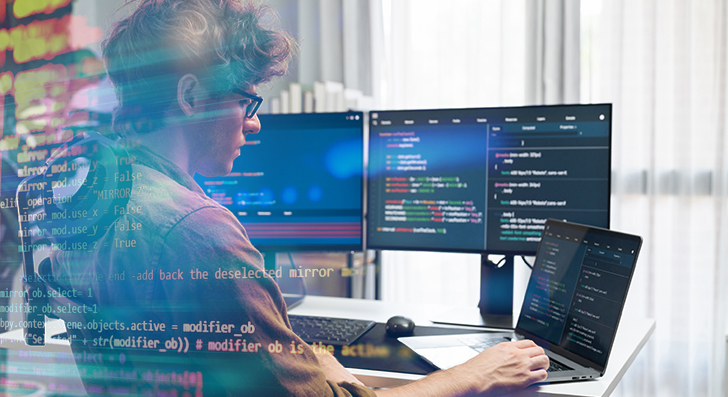
Scalability indicates your software can take care of development—much more buyers, additional info, and even more visitors—without breaking. For a developer, creating with scalability in your mind will save time and tension afterwards. Right here’s a transparent and functional manual to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your program from the start. Several purposes fall short every time they expand speedy due to the fact the first style and design can’t deal with the additional load. As a developer, you must think early about how your process will behave under pressure.
Commence by building your architecture for being versatile. Avoid monolithic codebases the place all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns split your application into lesser, independent elements. Just about every module or service can scale on its own with no influencing The complete method.
Also, think of your databases from working day a person. Will it require to deal with 1,000,000 end users or merely 100? Choose the proper form—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t require them but.
A different vital point is to avoid hardcoding assumptions. Don’t compose code that only operates underneath present-day conditions. Consider what would take place When your person foundation doubled tomorrow. Would your app crash? Would the database slow down?
Use layout designs that assist scaling, like message queues or celebration-pushed systems. These help your app deal with a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you're not just preparing for fulfillment—you might be cutting down long run complications. A properly-planned program is easier to maintain, adapt, and mature. It’s better to prepare early than to rebuild afterwards.
Use the proper Database
Choosing the right databases can be a vital part of developing scalable applications. Not all databases are built precisely the same, and using the Incorrect you can sluggish you down and even cause failures as your application grows.
Start off by knowing your information. Can it be very structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient match. They're strong with interactions, transactions, and regularity. In addition they aid scaling procedures like go through replicas, indexing, and partitioning to handle far more visitors and details.
If the information is a lot more versatile—like person action logs, merchandise catalogs, or documents—look at a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at handling substantial volumes of unstructured or semi-structured info and will scale horizontally a lot more conveniently.
Also, take into account your read and publish patterns. Are you presently undertaking lots of reads with much less writes? Use caching and read replicas. Will you be handling a heavy publish load? Explore databases that can take care of high generate throughput, and even party-primarily based details storage techniques like Apache Kafka (for non permanent info streams).
It’s also clever to think forward. You might not will need advanced scaling attributes now, but picking a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your details based on your accessibility designs. And often keep an eye on databases functionality while you increase.
Briefly, the appropriate databases will depend on your application’s framework, pace wants, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save many trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off adds up. Badly written code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular operates. Keep the features brief, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Future, have a look at your databases queries. These frequently gradual items down more than the code by itself. Make sure Every single question only asks for the information you truly require. Stay clear of Choose *, which fetches all the things, and as an alternative pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking a lot of joins, Particularly throughout significant tables.
Should you detect exactly the same knowledge remaining requested over and over, use caching. Retail store the results briefly working with applications like Redis or Memcached which means you don’t should repeat highly-priced operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may well crash whenever they have to manage one million.
To put it briefly, scalable apps are fast apps. Keep your code tight, your queries lean, and use caching when required. These measures support your software keep clean and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to a single server performing all the work, the load balancer routes buyers to unique servers determined by availability. This implies no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Other individuals. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for the identical information all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching minimizes databases load, improves pace, and will make your app additional effective.
Use caching for things which don’t change typically. And always be sure your cache is current when info does improve.
In brief, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app tackle much more end users, continue to be quick, and Get well from complications. If you plan to expand, you would like each.
Use Cloud and Container Equipment
To develop scalable applications, you'll need equipment that permit your application develop very easily. That’s wherever cloud platforms and containers are available. They give you flexibility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to invest in components or guess future capacity. When visitors raises, you'll be able to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection equipment. It is possible to target constructing your app rather than handling infrastructure.
Containers are another key Software. A container offers your app and every thing it must operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app concerning environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of one's application crashes, it restarts it mechanically.
Containers also allow it to be easy to individual elements of your application into providers. You can update or scale sections independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quick, deploy conveniently, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and assist you to remain centered on developing, not repairing.
Watch Every thing
In case you don’t observe your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of building scalable methods.
Start off by monitoring primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application far too. Regulate how much time it's going to take for buyers to load pages, how frequently errors happen, and where they happen. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for critical troubles. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes here down, you need to get notified instantly. This helps you fix challenges speedy, generally in advance of end users even recognize.
Monitoring is usually practical any time you make alterations. Should you deploy a brand new feature and find out a spike in problems or slowdowns, you are able to roll it again in advance of it triggers genuine destruction.
As your application grows, site visitors and data raise. With no monitoring, you’ll miss out on signs of hassle until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that increase smoothly without having breaking stressed. Start modest, Imagine large, and Make smart.